728x90
Animate With The iOS Keyboard In Swift
Create smooth layout animations when the iOS keyboard shows and hides.
www.advancedswift.com
Keyboard가 보이고 사라질 때, NSNotificationd의 userInfo에 들어있는 필드들을 통해서 keyboard의 크기와 animation duration, curve정보까지 받아낼 수 있다.
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var textField: UITextField!
@IBOutlet weak var labelTopMargin: NSLayoutConstraint!
let defaultTopMargin: CGFloat = 500
override func viewDidLoad() {
super.viewDidLoad()
labelTopMargin.constant = defaultTopMargin
// Subscribe to Keyboard Will Show notifications
NotificationCenter.default.addObserver(self, selector: #selector(keyboardWillShow(_:)), name: UIResponder.keyboardWillShowNotification, object: nil)
// Subscribe to Keyboard Will Hide notifications
NotificationCenter.default.addObserver(self, selector: #selector(keyboardWillHide(_:)), name: UIResponder.keyboardWillHideNotification, object: nil)
self.view.addGestureRecognizer(UITapGestureRecognizer(target: self, action: #selector(hideKeyboard)))
}
@objc
func hideKeyboard() {
textField.resignFirstResponder()
}
@objc
dynamic func keyboardWillShow(_ notification: NSNotification) {
animateLabelWithKeyboard(notification: notification)
}
@objc
dynamic func keyboardWillHide(_ notification: NSNotification) {
animateLabelWithKeyboard(notification: notification)
}
func animateLabelWithKeyboard(notification: NSNotification) {
animateWithKeyboard(notification: notification, animations: { keyboard in
self.labelTopMargin.constant = self.defaultTopMargin - (UIScreen.main.bounds.height - keyboard.origin.y)
})
}
}
extension ViewController {
func animateWithKeyboard(notification: NSNotification, animations: ((_ keyboardFrame: CGRect) -> Void)?) {
// Extract the duration of the keyboard animation
let durationKey = UIResponder.keyboardAnimationDurationUserInfoKey
let duration = notification.userInfo![durationKey] as! Double
// Extract the final frame of the keyboard
let frameKey = UIResponder.keyboardFrameEndUserInfoKey
let keyboardFrameValue = notification.userInfo![frameKey] as! NSValue
// Extract the curve of the iOS keyboard animation
let curveKey = UIResponder.keyboardAnimationCurveUserInfoKey
let curveValue = notification.userInfo![curveKey] as! Int
let curve = UIView.AnimationCurve(rawValue: curveValue)!
// Create a property animator to manage the animation
let animator = UIViewPropertyAnimator(
duration: duration,
curve: curve
) {
// Perform the necessary animation layout updates
animations?(keyboardFrameValue.cgRectValue)
// Required to trigger NSLayoutConstraint changes
// to animate
self.view?.layoutIfNeeded()
}
// Start the animation
animator.startAnimation()
}
}
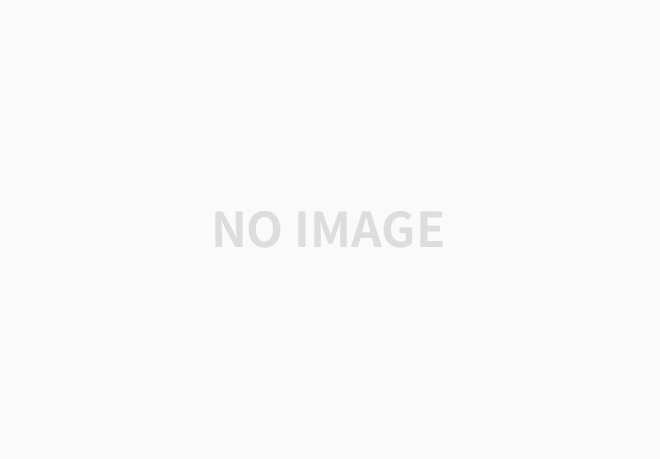
728x90
'iOS > 설명' 카테고리의 다른 글
[iOS] iOS에서의 대표적인 Design Pattern들 (0) | 2023.01.21 |
---|---|
[iOS] nibName에 따른 ViewController 초기화 (0) | 2023.01.21 |
[iOS] 앱 실행 방법에 따른 AppDelegate 호출 메서드 (0) | 2023.01.07 |
[iOS] CALayer.shouldRasterize (0) | 2023.01.01 |
[iOS] 앱의 샌드박스 구조 (0) | 2022.12.31 |
댓글