이제 초기화 메소드들은 전부 작성했으니 그 다음 user가 사용할 메소드들을 작성하기로 합니다.
5. 스케줄 관련 기능
우선 시간표이니 스케줄과 관련된 기능들이 있어야 될테니 맨처음 addSchedule()을 작성했습니다.
//스케줄 추가
public void addSchedule(String text, String row_name, String column_name, int blocks)
{
//스케줄 추가 cell
Cell schedule_cell = findCell(row_name,column_name);
if(schedule_cell.getVisibility() == View.GONE || schedule_cell.isScheduled())//스케줄 있는 시간
{
Toast.makeText(getContext(),"already exists schedule",Toast.LENGTH_SHORT).show();
return;
}
ArrayList<String> row_list = new ArrayList<>(Arrays.asList(row_names));
int origin_index = row_list.indexOf(row_name);
//cell 삭제
for(int i=1;i<blocks;i++)
{
Cell cell= findCell(row_names[origin_index+i],column_name);
cell.setVisibility(View.GONE);
schedule_cell.addSpannedCells(cell);
}
schedule_cell.setText(text);
schedule_cell.setScheduled(true);
schedule_cell.setClickable(true);
//병합
GridLayout.LayoutParams layoutParams = (GridLayout.LayoutParams)schedule_cell.getLayoutParams();
layoutParams.rowSpec = GridLayout.spec(origin_index, blocks,1.0f);
schedule_cell.setLayoutParams(layoutParams);
}
스케줄 추가 기능은
'스케줄 있는 시간인지 확인' -> '없으면 차지하는 block수만큼 밑에 있는 cell들을 View.GONE 처리 시키기' -> 'span 기능으로 병합'
으로 이루어집니다.
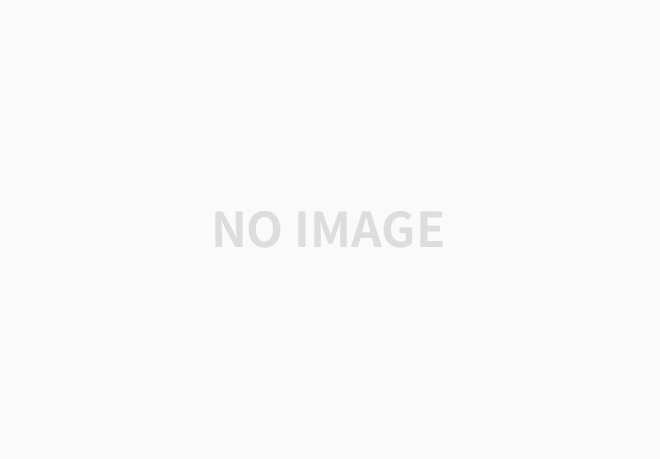
만약 운영체제 스케줄을 추가하고 싶으면
addSchedule("운영체제","1교시","수",2,getResources().getColor(R.color.red,null), getResources().getColor(R.color.white,null));
라고 적으면 됩니다. blocks는 몇칸 차지하는지를 나타내는 것입니다. 운영체제 시간은 1교시 2교시 총 2칸 차지하니까 2를 입력하면 됩니다.
다음 deleteSchedule
public void deleteSchedule(String row_name, String column_name)
{
Cell schedule_cell = findCell(row_name, column_name);
int row = schedule_cell.getRow();
if(!schedule_cell.isScheduled())//스케줄 없는경우
{
Toast.makeText(getContext(),"not exists any schedule",Toast.LENGTH_SHORT).show();
return;
}
//스케줄 삭제처리
schedule_cell.setText("");
schedule_cell.setScheduled(false);//스케줄 삭제됨
schedule_cell.setClickable(false);
//색 복구
schedule_cell.setBackgroundColor(cellColor);
schedule_cell.setTextColor(cellTextColor);
//병합 복구
GridLayout.LayoutParams layoutParams = (GridLayout.LayoutParams)schedule_cell.getLayoutParams();
layoutParams.rowSpec = GridLayout.spec(row, 1,1.0f);
schedule_cell.setLayoutParams(layoutParams);
//지워진 cell들 원래대로
ArrayList<Cell> spannedCells = schedule_cell.getSpannedCells();
for(int i=0;i<spannedCells.size();i++)
{
spannedCells.get(i).setVisibility(View.VISIBLE);
}
spannedCells.clear();
}
원래 있던 스케줄 텍스트를 지우고 cell 배경색과 텍스트 색깔 복구, 병합되어서 View.GONE처리 되었던 cell들을 다시 visible 처리합니다.
스케줄 수정도 가능해야 되니깐
public void modifySchedule(String row_name, String column_name, String change_text, int blocks)
{
Cell schedule_cell = findCell(row_name, column_name);
int row = schedule_cell.getRow();
int column = schedule_cell.getColumn();
if(!schedule_cell.isScheduled())//스케줄 없는경우
{
Toast.makeText(getContext(),"not exists any schedule",Toast.LENGTH_SHORT).show();
return;
}
schedule_cell.setText(change_text);
ArrayList<Cell> spannedCells = schedule_cell.getSpannedCells();
for(int i=0;i<spannedCells.size();i++)
{
spannedCells.get(i).setVisibility(View.VISIBLE);
}
spannedCells.clear();
//cell 삭제
for(int i=1;i<blocks;i++)
{
Cell cell= findCell(row + i,column);
cell.setVisibility(View.GONE);
schedule_cell.addSpannedCells(cell);
}
//병합
GridLayout.LayoutParams layoutParams = (GridLayout.LayoutParams)schedule_cell.getLayoutParams();
layoutParams.rowSpec = GridLayout.spec(row, blocks,1.0f);
schedule_cell.setLayoutParams(layoutParams);
}
이건 그냥 deleteSchedule -> addSchedule입니다.
6. cell 관련 기능
이제 cell을 커스텀 하는 기능들도 있어야 합니다. 제일 기본적인 것은 모든 cell view들을 불러 오는 기능이죠.
//모든 cell 가져옴
public Cell[][] getAllCell()
{
Cell[][] list = new Cell[getRowCount()] [getColumnCount()];
for(int i=0;i<getRowCount();i++)
{
for(int j=0;j<getColumnCount();j++)
list[i][j] = findCell(row_names[i],column_names[j]);
}
return list;
}
그리고 전체 cell의 textcolor 변경
//cell 배경 색
public void setCellColor(int cellColor) {
this.cellColor = cellColor;
for(int i=0;i<getRowCount();i++)
{
for(int j=0;j<getColumnCount();j++)
{
Cell cell = (Cell) findViewWithTag(row_names[i]+"-"+column_names[j]);
cell.setBackgroundColor(cellColor);//배경설정
}
}
}
cell간 마진값 조정 기능(마진이 0이 되버리면 간격 구분이 안되기에 기본적으로 5로 설정해놨어요)
//마진 값
public void setCellsMargin(int left, int top, int right, int bottom)
{
cellMarginLeft = left;
cellMarginRight = right;
cellMarginTop = top;
cellMarginBottm = bottom;
for(int i=0;i<getRowCount();i++)
{
for(int j=0;j<getColumnCount();j++)
{
Cell cell = (Cell)findViewWithTag(row_names[i]+"-"+column_names[j]);
GridLayout.LayoutParams layoutParams = (GridLayout.LayoutParams)cell.getLayoutParams();
layoutParams.setMargins(left,top,right,bottom);
cell.setLayoutParams(layoutParams);
}
}
}
그리고 특정 cell을 찾아오는 기능
//특정 row, column의 cell 반환
public Cell findCell(String row_name, String column_name)
{
Cell cell = (Cell)findViewWithTag(row_name+"-"+column_name);
return cell;
}
//특정 row, column의 cell 반환
public Cell findCell(int row, int column)
{
Cell cell = (Cell)findViewWithTag(row_names[row]+"-"+column_names[column]);
return cell;
}
//해당 cell의 text를 보고 찾음
public Cell findCellWithText(String text)
{
for(int i=0;i<getRowCount();i++)
{
for(int j=0;j<getColumnCount();j++)
{
Cell cell = (Cell)findViewWithTag(row_names[i]+"-"+column_names[j]);
if(cell.getText().equals(text))
return cell;
}
}
return null;
}
오버로딩을 통해 정수값 혹은 미리 설정해놓은 string 값 어떤 것이든 다 사용해서 가져올 수 있게 했습니다. schedule의 text로도 찾게 해놓았어요.
일단 현재까지 기능들은 이렇게만 추가를 해놓았고 아직 어떤 기능을 더 추가할지 생각을 하는 중인데 아마 애니메이션을 적용해보지 않을가 싶습니다. 추가 기능을 적용하면 다시 포스팅 해보도록 하겠습니다.
'Project' 카테고리의 다른 글
[프로젝트] 모이소 : PC 멀티리모콘 앱 개발기 - 1 (0) | 2020.08.29 |
---|---|
[프로젝트] 모이소 PC 앱 배포 (Beta) (0) | 2020.08.28 |
[프로젝트] 대학시간표(CollegeTimeTable) 레이아웃 커스텀 뷰 제작기 - 4 (0) | 2020.08.28 |
[프로젝트] 대학시간표(CollegeTimeTable) 레이아웃 커스텀 뷰 제작기 - 3 (0) | 2020.08.28 |
[프로젝트] 대학시간표(CollegeTimeTable) 레이아웃 커스텀 뷰 제작기 - 1 (3) | 2020.08.28 |
댓글